3.1. About Python#
Interactive page
This is an interactive book page. To solve quizzes and run Python code cells, press launch button at the top right side of the page (look for a small rocket icon) and then Live Code.
3.1.1. Interacting with Python#
There are various ways in which you can interact with Python language and run Python code:
Terminal. You can create and edit Python scripts using a text editor such as Notepad or Vim (because Python scripts are text files), and then run them from the terminal.
IDE. IDEs are comprehensive development environments which you can use to write, test, and debug your code. They can also be linked to Git for version control and offer a set of useful features that can help you with programming. We recommend using IDE called Visual Studio Code (VS Code) alongside this book’s materials.
This book. In this book, we offer interactive pages which, once launched, contain interactive Python coding cells. This page is also an interactive page. You can use in-book Python coding cells while learning and exploring new concepts. However, when working on larger pieces of code, such as exercises from this book, you are encouraged to do it in IDE.
Jupyter notebooks. Notebooks are another popular way to interact with Python. They provide an interactive programming environment, in which you can incorporate text and blocks/cells of code. If you’re curious to learn more, take a look at this quick start guide.
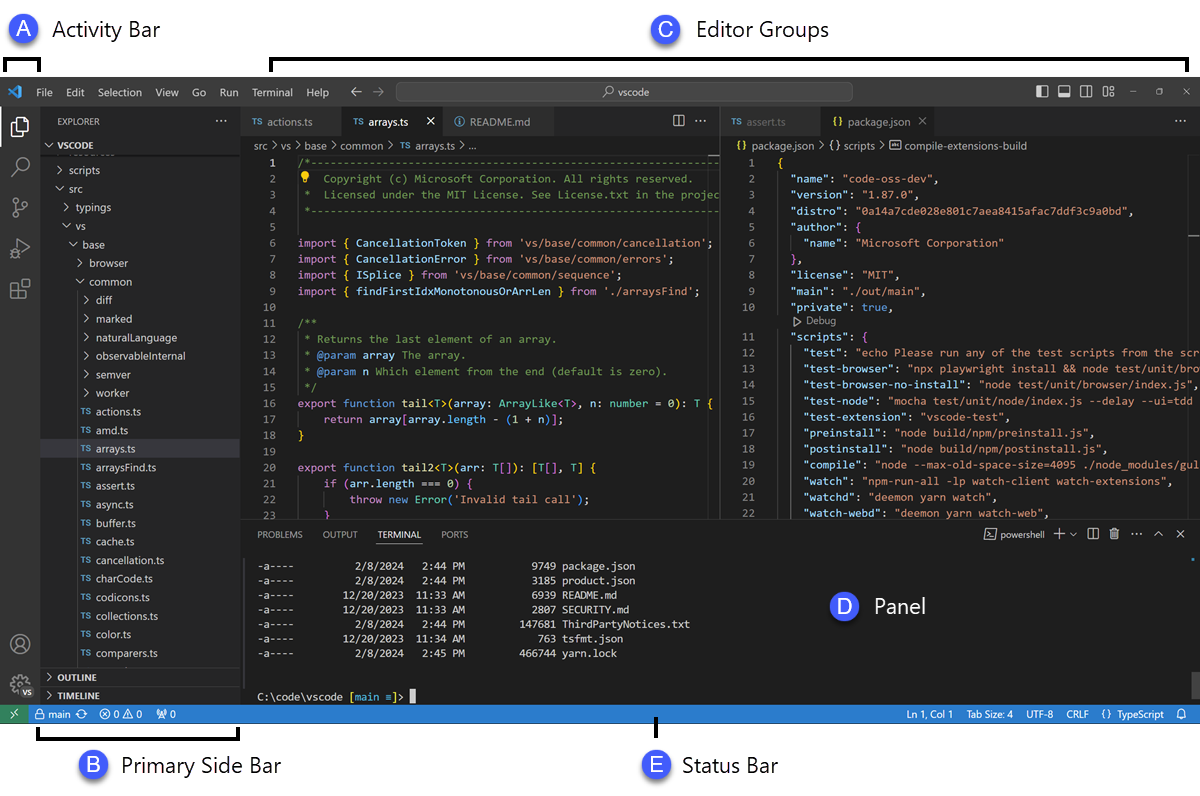
Fig. 3.2 VS Code interface. We will install VS Code and walk you through its usage in the subsequent pages. You can also visit VS Code website to learn more.#
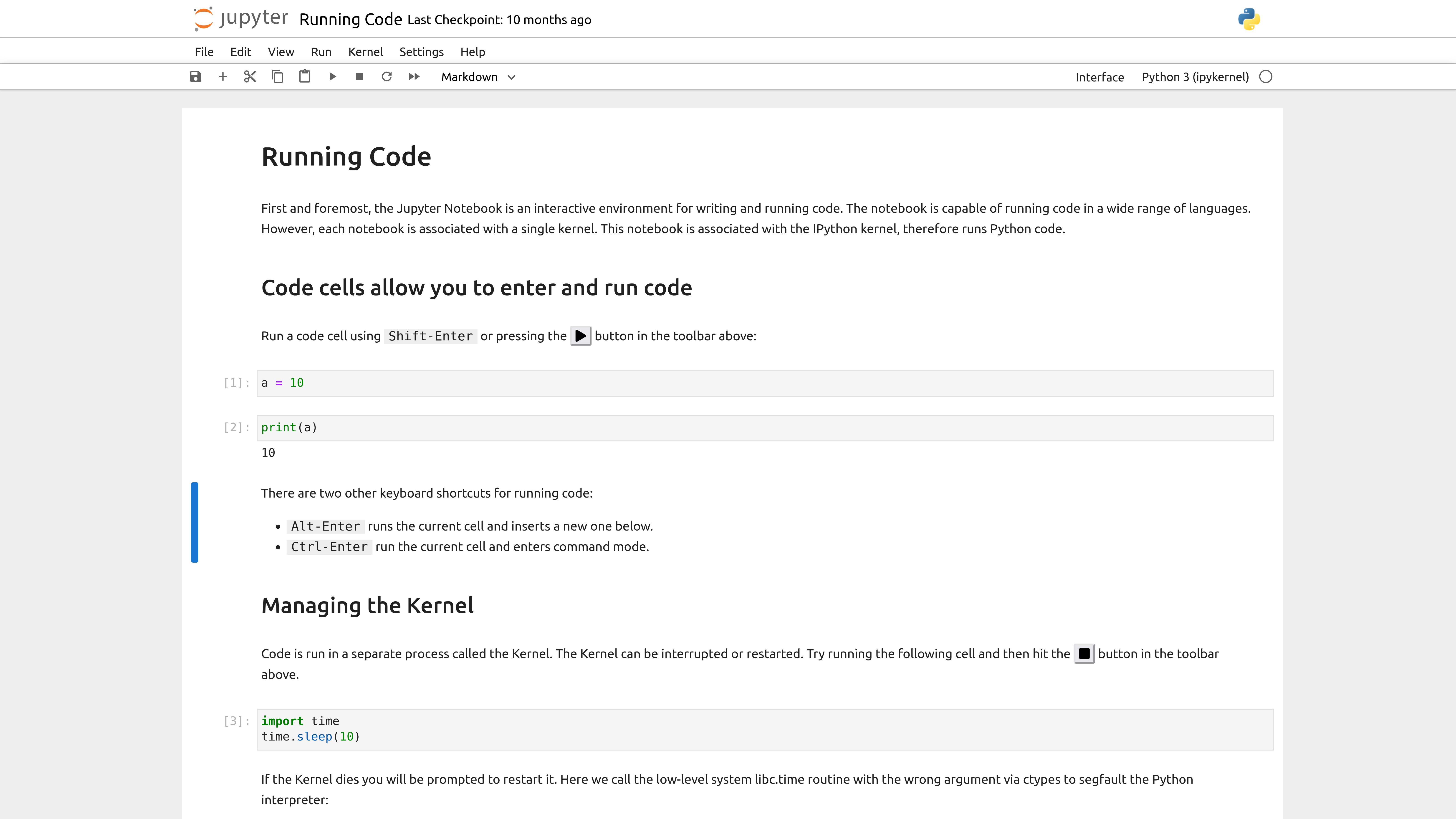
Fig. 3.3 Jupyter notebook interface. To learn more, visit Jupyter notebook website.#
3.1.2. An interpreted language#
Python is an interpreted computer programming language. Every time you run Python (either in the command line, in an IDE such as VS Code, or in a Jupyter notebook), a Python kernel is created. This kernel is a copy of the Python program (interpreter) that runs on your computer. You can interact with and give commands to the kernel by typing Python commands in VS Code (or a code cell in a Jupyter notebook).
Interactive code cells are also included in this book, allowing you to run Python code from within this book.
For example, the cell below contains Python code that prints the text Hello world!
(a classical way to start learning programming).
To run this Python code cell, press run
.
Remember that you have to launch a live version of the page to run Python code.
What output do you get when you run
this code?
Can you make the code print out something else, such as your name?
print("Hello world!")
When you run the cell, the code will be sent to the Python kernel, which will translate your command into a binary language your computer CPU understands, send it to the CPU, and read back the answer. If the code you run produces an output, meaning that the kernel will send something back to you (in the above example, the printed text), then the output that your code produces will be displayed in the VS Code terminal (or below the code cell here in the book).
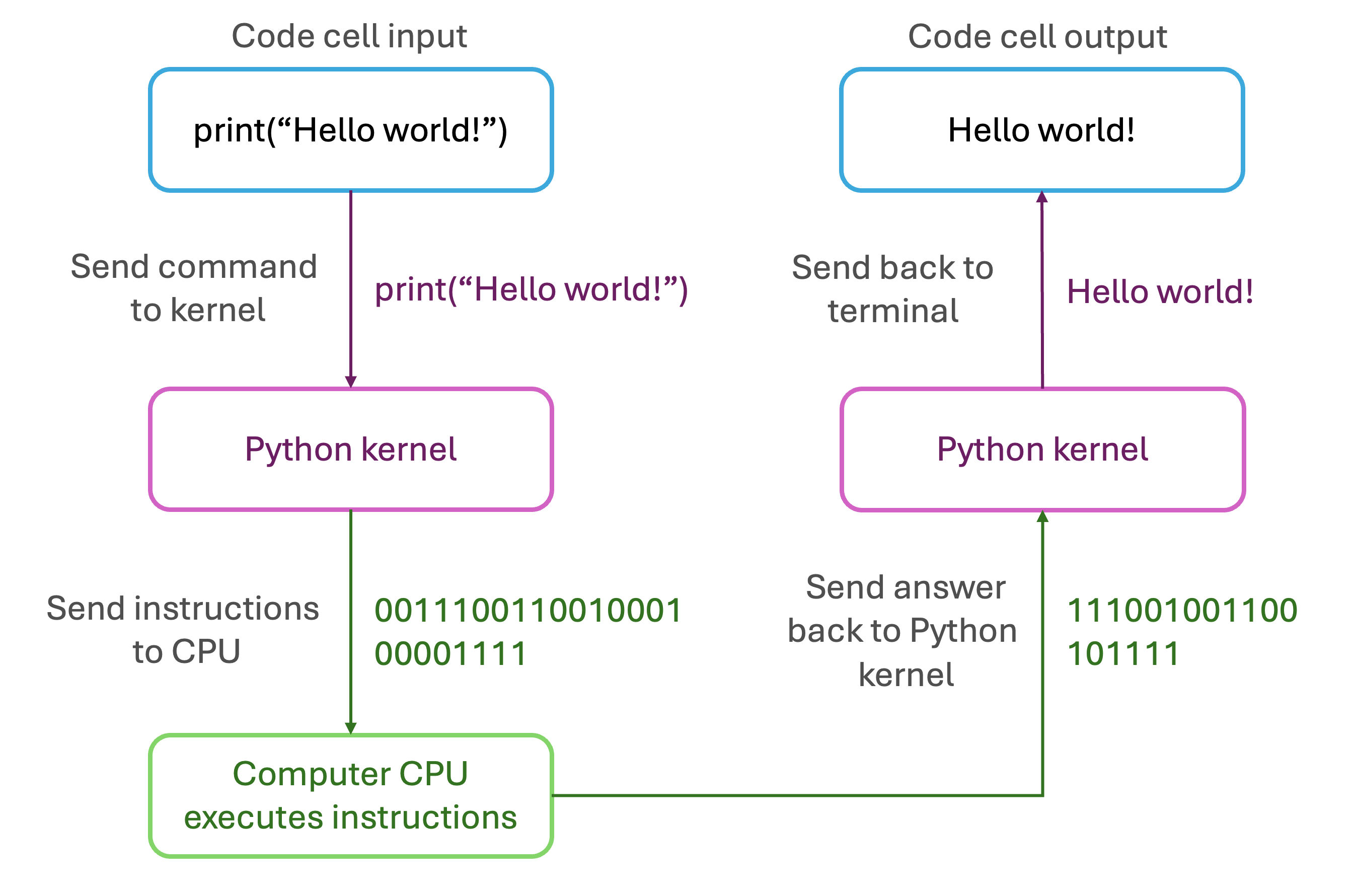
Fig. 3.4 Scheme of what running a Python code looks like “behind the scenes”.#
Your code can of course contain multiple lines and commands.
In addition, it is good practice to add comments to your code to explain what it’s doing.
This makes your code more readable both to yourself and others, and it’s good practice to comment your code (see section on good coding pratices).
Starting a line with a hashtag #
tells Python to ignore the line when executing code, so we use #
for writing comments.
# This is a line of comments and it is not executed
# Comments are amazing for explaining what the code does
# The line below prints a message that greets the world
print("Hello world!")
print("Goodbye")
Compiled and interpreted languages
On the basis of how a programming language is processed, we make a distinction between compiled and interpreted languages. Python belongs to the latter group.
Compiled language source code has to be compiled into machine code (binary language), which can then be executed by your computer’s CPUs. IDEs have built-in compilers that can do this for you. While compiled language code is faster because it gets translated into machine code prior to execution, it is also less portable because it’s optimized for specific hardware and OS on which it is meant to run. Examples of compiled languages include C, C++, and Java.
For interpreted languages, the source code is read and executed line by line directly by an interpreter. In other words, the interpreter translates code into machine code at runtime, directly in an IDE or command line, and there is no separate compilation step. Translation at runtime makes interpreted languages slower, but also more portable. Examples of interpreted languages include Python, JavaScript, and Ruby.
3.1.3. Natural vs. programming language#
While Python may resemble plain English, there are still crucial differences between natural languages (such as English) and programming languages (such as Python).
Programming languages have very strict syntax and there is no tolerance for errors - even a small syntax error may prevent your Python code from running and must be corrected. Note that this is analogous to other fields of science:
Field |
Correct syntax |
Incorrect syntax |
---|---|---|
Mathematics |
3 + 3 = 6 |
3 = +6* |
Chemistry |
H2O |
2XeMg |
Python |
print(“Hello world!”) |
“print(Hello world!”) |
Let’s see this in practice with print()
that we used above. In Python, when we want to print some text, such as “Hello world!”, the correct syntax is using the print
statement with round brackets, and the text inside the brackets has to be in quotation marks.
What happens if one or both quotations marks are missing or misplaced? What happens if you have a typo in print
, e.g., prit
?
Use the code cell below to introduce mistakes into Python’s print
statement and try to run the code with mistakes.
print("Hello world!")
3.1.4. Python kernel has memory#
In addition to having Python do things for you, such as printing Hello world!
, you can also make Python kernel remember things for you.
Let’s say you want Python to remember number 5 for you.
You can execute the following line:
a = 5
In Python, the =
symbol represents the assignment operator: it is an instruction to assign the value of 5 to the variable called a
.
If a
already exists, it will be over-written with the new value; if variable a
does not yet exist, then Python will create a new variable for you automatically.
Therefore, the cell above created variable a
that has the value of 5 in the memory of the Python kernel.
We can check that a
is indeed remembered by now printing its value:
print(a)
Warning
The equation sign can have different meanings in Python, depending on how you use it:
=
is the assignment operator: it assigns values to variables. Runninga = 5
will assign 5 toa
.==
is the equality operator: it compares operands for equality. If we have defineda
to be 5, runninga == 5
will return a booleanTrue
. We will learn more about the==
operator in a later section on Flow control.
Besides numerical values, variables can also be strings, which are sequences of characters. You make a string by putting the text between the quotation marks. Can you guess what the printed output of the following command will be?
print("a")
Note that we can also combine several variables, or strings and a variable, within one print
statement using a comma ,
.
This can be used to add a message, i.e., some context to the variables you’re printing.
print("My favourite number is", a)
Exercise 3.1
Write a Python code containing two lines.
In the first line, assign the numerical value 3.14 to a variable called
pi
.In the second line, use a
print
statement to print out the value of your variable. Add a descriptive string inprint
(e.g., “The approximate value of pi is”).Can you also use special symbols in strings, e.g., if you were to write “The approximate value of pi =”?
# Use this cell to write your code
To combine multiple types of variables in a string or in a print
statement, we can also use formatted string (f-strings). These usually make your code a bit more readable. To create an f-string, we preface the string with an f (f".."
) and we put the variables between curly brackets ({variable}
). For example:
plant = "Arapidopsis thaliana"
human = "Homo sapiens"
plant_chr = 5
human_chr = 23
print(f"Plan {plant} has {plant_chr} chromosomes, which is much less than human {human} with {human_chr} pairs of chromosomes.")