2.2. BASH commands#
Interactive page
This is an interactive book page. To solve quizzes, press the launch button at the top right side of the page (look for a small rocket icon) and then Live Code.
To begin familiarizing yourself with the terminal, we first provide an overview of commonly used BASH commands (Table 2.1), which are explained in more detail below on this page. The overview is not exhaustive - if you want to perform an action not covered by the table, search online to find an appropriate command. To run a command on your computer, simply open your terminal, type in the command, and press Enter.
Command |
Effect |
Details |
---|---|---|
|
Print working directory |
Display the location (path) of the directory in which you’re currently working. |
|
Change directory |
Navigate between directories. To go to the home directory, use |
|
Make a new directory |
Creates a new empty directory with the user-defined name. |
|
Remove a directory |
Deletes a directory if it’s empty. See also |
|
List contents of a directory |
Use with |
|
Open a file to view its contents. To exit an opened file, press |
Doesn’t allow for file editing. |
|
Print file contents to terminal |
If used with more than one file, it concatenates them and prints the result in the terminal. |
|
Copy |
Copy a file to another directory, or make a file copy in the same location. To copy a directory with all its contents, use |
|
Move / Rename |
Moves or renames files. Renames directories. To move directories with their contents, use |
|
Remove |
Deletes a file. |
|
Search |
Search for text patterns. |
|
Clear terminal window |
Clears commands and outputs to declutter the terminal. |
|
Current folder |
|
|
One folder up (parent folder) |
|
|
Wildcard |
Replaces any text. |
|
Redirect |
Instead of printing output of running a script in the terminal, with |
Online information
Don’t hesitate to look for information online (Google, StackOverflow, ChatGPT) when you’re not sure how to do something, or what is the exact command that you need to use.
2.2.2. Manipulating directories#
With the terminal you can easily create (mkdir A/
) or delete an empty directory A/
(rmdir A/
), where A/
can be something like Movies/
in the figure above.
You can also use rm
to delete directories, cp
to copy, and mv
to move and rename them.
If you have a directory with files inside (i.e, a non-empty directory), you cannot use rmdir
to delete it. In order to delete a non-empty directory with all its contents, you need to use rm
in a recursive fashion: rm -r A/
.
Similarly, if you wish to make a copy of a directory (with all its contents), this also has to be done recursively. Notice the nuanced differences between the following commands:
cp -r A/ new_A/
- makes a copy of the source directoryA/
with all its contents; the new folder is callednew_A/
.cp -r A new_A/
- same as previous command.cp -r A/ existing_A/
- copies the contents of the source directoryA/
to the existing destination directoryexisting_A
.cp -r A existing_A/
- makes a copy of the directoryA/
with all its contents inside the existing directoryexisting_A
. Therefore, the presence or absence of the trailing slash/
in the source directory determines whether the entire directory is copied as a subdirectory or only its contents are copied.
Note: in Cygwin, there is no difference between the latter two commands, but in macOS/Linux terminal there is.
In addition to copying, we can also move directories.
To move directory A/
with all its contents into directory B/
, we can use mv A/ B/
(note: mv
doesn’t differentiate based on the presence or absence of a trailing slash in the source directory).
We can also use mv
to rename a directory like this: mv A/ new_name_A/
.
Exercise 2.4
Try to use rm
with one of your non-empty folders. What message does the terminal return?
Exercise 2.5
In your terminal:
Navigate to your desired working directory (this could be, e.g.,
Documents/
orDesktop/
).Create two new directories: name them
A
andexisting_A
.Copy any file to directory
A/
. Because we don’t yet know how to do it in the terminal, you can do it using your mouse and GUI.From your working directory, try to run the
cp
andmv
commands mentioned above and observe what the effects are. Note: you may need to “reset” your setup with two folders depending on which command you ran.
Recalling previous commands
Sometimes you want to use the same command you used recently in your terminal, either to run it again, or make a small modification and then run it.
There’s an easy way to fetch your previously executed commands instead of retyping them: you can simply press arrow up (↑) once or multiple times.
To print a full list of recent commands, use history
command.
Try it in your terminal!
2.2.3. Working with files#
Several commands that apply to working with directories also apply to files. Let’s take a look at what the following commands mean:
cp a.txt b.txt
- copiesa.txt
tob.txt
in the same directory. Usingmv
instead ofcp
would renamea.txt
intob.txt
.cp a.txt ../
- copiesa.txt
to one directory up (it can also be used withmv
to move the file).cp a.txt A/
- as previous command, but copiesa.txt
in the directoryA/
(can also be used withmv
).cp ../a.txt .
- copiesa.txt
from one directory up to the current (.
) directory (it can also be used withmv
).
2.2.3.1. Wildcard#
Sometimes you will want to copy or move multiple files or directories at the same time.
To avoid typing each command individually or recalling the same command and changing it multiple times, you can use the wildcard *
to replace any text.
For instance, let’s say we are located in the directory Physics
:
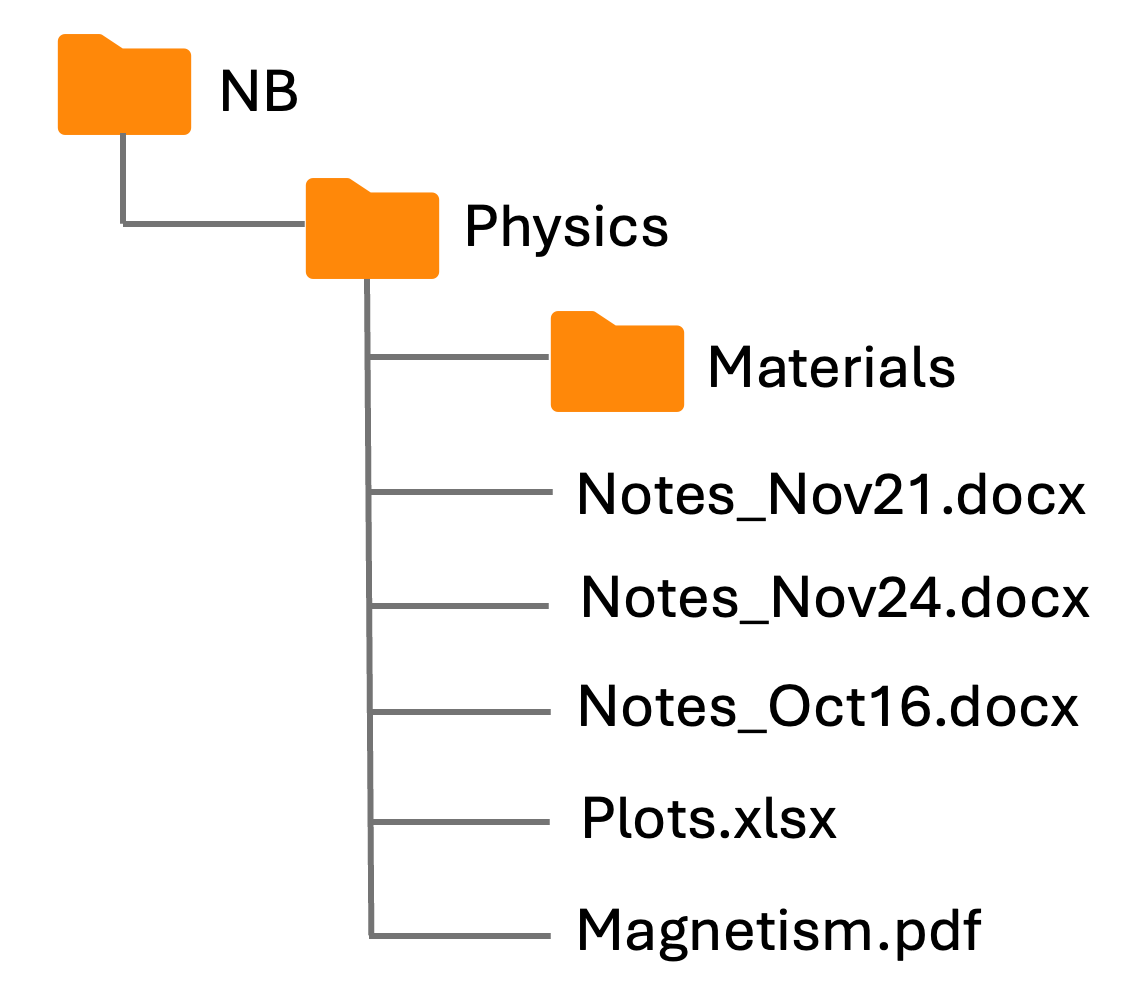
Fig. 2.3 Example folder: wildcard usage.#
If we are in the directory Physics
and want to move all Notes files into directory Materials/
, we could use mv Notes* Materials/
.
With Notes*
, we included all three .docx
files. Therefore, *
effectively means Nov21.docx
or Nov24.docx
or Oct16.docx
.
We could alternatively use mv N* Materials/
because our three files with notes are the only files beginning with letter N, so mv N*
already defines our selection. Another option would be to use mv *docx Materials/
because Notes are the only .docx
files in the directory Physics/
.
Note that it’s also possible to use multiple wildcards in one go if there are several patterns you wish to generalize. E.g., to delete all .docx
files that have Nov in their name, you can use rm *Nov*.docx
.
import micropip
await micropip.install("jupyterquiz")
from jupyterquiz import display_quiz
import json
with open("question2.json", "r") as file:
questions=json.load(file)
display_quiz(questions, border_radius=0)
2.2.3.2. Reading files#
For taking a glance at the contents of a text file in read-only mode, less
comes in very handy.
To open a file for reading, we use less file.txt
.
We can further navigate through the text of a file by scrolling or search for specific text patterns like this: /Pattern
(note that this is case sensitive, i.e., there is a difference between /Pattern
and /pattern
).
To navigate to the end of the file, use G
. To move to the beginning of the file, use g
.
To close the file, simply press q
(for “quit”) on your keyboard.
An alternative command used to read the contents of a file is cat
.
Unlike the less
command which opens a file, cat
prints a file’s contents to your terminal.
If you use it with more than one file, e.g., cat fileA.txt fileB.txt
, it will concatenate the files and print the output in the terminal.
2.2.3.3. Editing files with Vim#
Vim is a powerful text editor which you can use from within the terminal.
Unlike text editors such as Notepad or MS Word, Vim is entirely command-based.
That means that even simply writing some new text in the file requires extra steps instead of just starting to type (concretely, you have to press i
to let Vim know that you wish to type new text).
Upside: you can’t accidentally add new text, which is actually quite useful when working with coding and scripts.
Vim does take some getting used to, but is ultimately a powerful text editor and usually comes pre-installed (e.g., when you remotely connect to a supercomputing cluster such as DelftBlue).
2.2.3.3.1. Opening and closing files#
To open a file in Vim, use:
vim filename.txt
If filename.txt exists, it will open for editing. If it does not exist, a new file with that name will be created.
There are several options to exit Vim:
:q → quit (only works if no changes were made)
:q! → quit without saving
:wq → save and quit
Exercise 2.6
Try out the commands for opening and closing files in Vim in your terminal. You can use a new or an existing file.
2.2.3.3.2. Vim’s modes#
Unlike standard text editors, Vim operates in different modes:
Normal mode (default) – used for navigation, copying, pasting, deleting, and running commands.
Insert mode (activated with
i
) – used for typing text.Visual mode (activated with
v
orV
) – used for selecting text. See more here.Command mode (activated with
:
) – used for saving, quitting, and running advanced commands.
You can also switch between modes when you work on a file. To do so:
Press
i
to enter insert modePress
Esc
to return to normal modeUse
:
to enter command mode
Command |
Effect |
Details |
---|---|---|
|
Insert text |
Puts Vim in insert mode, allowing text entry. |
|
Exit insert mode |
Return to normal mode. |
|
Undo |
Reverts the last change. |
|
Redo |
Re-applies an undone change. |
|
Delete line |
Removoes the current line. Use |
|
Delete a character |
Deletes a character at the position of the cursor. |
|
Copy-paste |
|
|
Replace |
Replaces occurrences of |
|
Search for text |
Finds the next occurrence of |
|
Beginning |
|
|
End |
|
|
Move cursor |
Left, down, up, and right, respectively. |
|
Show line numbers |
Displays line numbers on the left. |
|
Enter visual mode |
Enables text selection for copy/paste. |
|
Visual Line Mode |
Selects whole lines instead of individual characters. |
|
Macro |
Allows for defining custom macros (think of those as functions). |
|
Save file |
Saves changes without quitting. |
|
Close file |
|
Exercise 2.7
Open a terminal and navigate to your desired directory. Create and open a new file with vim my_file.txt
. Press i
to enter insert mode. Type a few lines of text, making sure they contain the following words: “word” and “foo”. Press Esc
to exit insert mode. Save the file and exit using :wq
. Reopen the file in Vim or with less
to confirm your changes.
Exercise 2.8
Open my_file.txt
from the previous exercise in Vim. Use these commands to experiment:
yy
to copy a line,p
to paste it.dd
to delete a line./word
to search for “word” in the file.:%s/foo/bar/gc
to replace “foo” with “bar”. When you’re done, save and exit.
Advanced example: using macros
Vim allows for automating repetitive tasks using macros.
Let’s consider the following task: Replace every occurrence of “A” with “B” in 1,000 consecutive lines. To do this in Vim, we can build a macro using these steps:
Position your cursor on the first “A”.
Start recording a macro with
qa
→q
starts recording, anda
is the macro name.Type
i B Esc j
→i
enters insert mode,B
replaces “A” with “B”,Esc
exits insert mode,j
moves cursor down one line.Stop recording with
q
.Run the macro 1000 times with
1000@a
.
This automates the task efficiently!
import micropip
await micropip.install("jupyterquiz")
from jupyterquiz import display_quiz
import json
with open("question3.json", "r") as file:
questions=json.load(file)
display_quiz(questions, border_radius=0)