1.1. Computer glossary#
A glossary with some of the computer terms used throughout the book. You can choose to either read them in detail to learn what these terms mean, skim over them, or skip this page altogether and return at a later point in time. Most of the terms will also be explained later in the book, so you can think of this page as an overview place.
You will notice, both here and throughout this book, that some terms are not unique to the computational world (e.g., function or library). Therefore, a part of the learning process will be to understand the meaning of the words within a given context.
Programming
Programming is a set of instructions that allows the computer to automate a task or solve a problem.
Algorithm
An algorithm is a step-by-step procedure for solving a problem using computer code. In other words, with an algorithm you are sketching what your approach to solving a problem will be. It is useful to think of the steps that will be needed before starting to write your code.
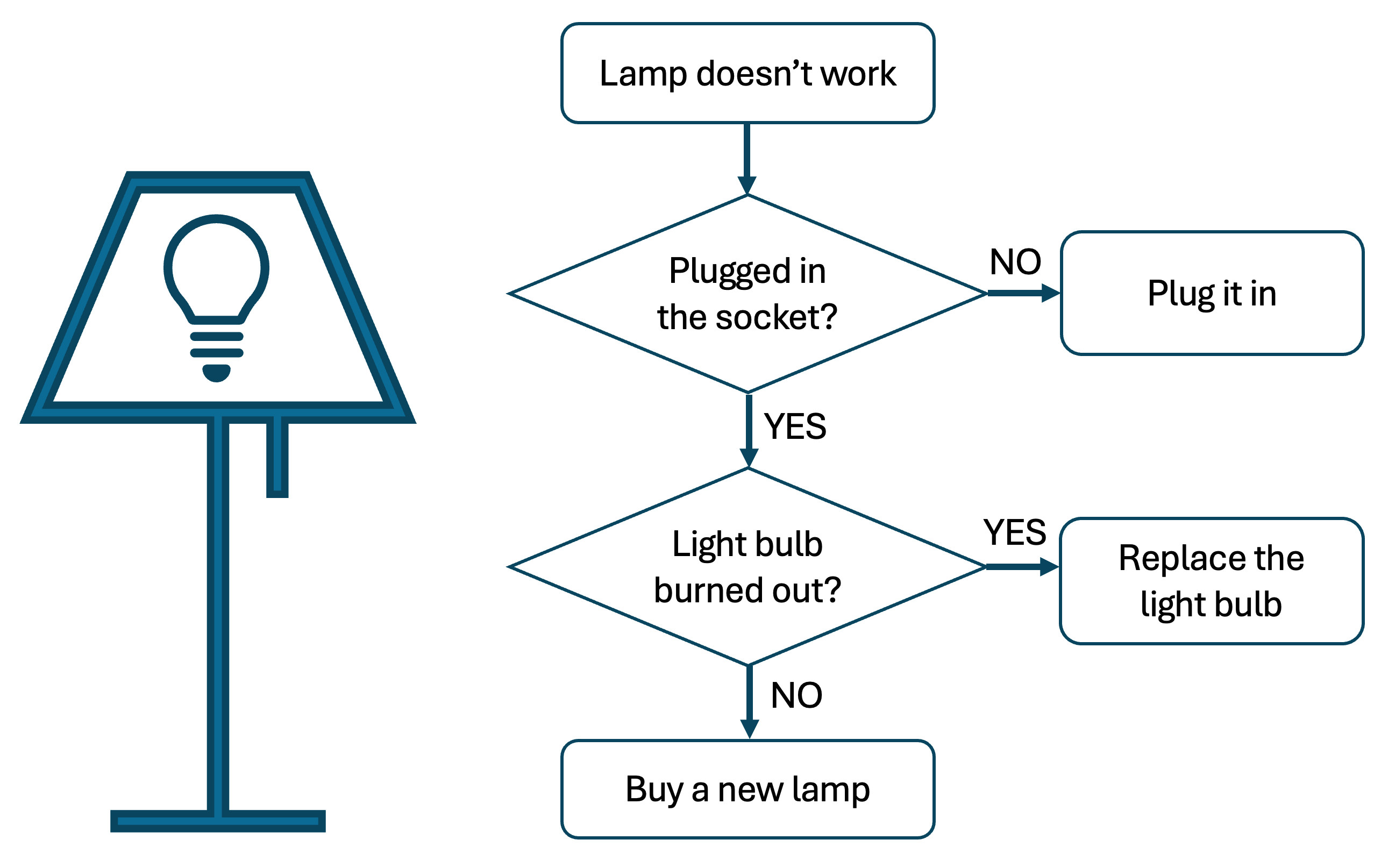
Fig. 1.2 Scheme of an algorithm for solving the problem of lamp not working.#
Computer program and code
Computer program is an implementation of computer code which instructs the computer how to execute the algorithm.
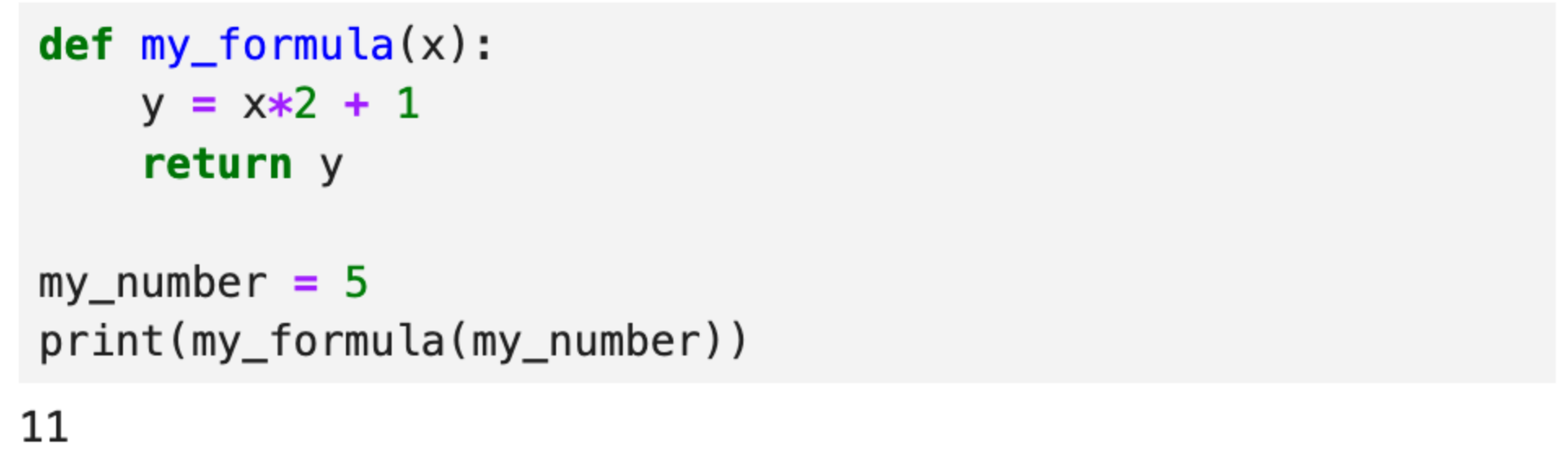
Fig. 1.3 Python code that defines \(my\_function\) and prints the output of passing \(my\_number\) to the function (2*5+1 = 11).#
Programming language and syntax
To write the instructions in such as way that a computer can understand them, i.e., to write a code, we use a programming language. Python, which we will use in this course, is an example of a computer language. While there are thousands of languages developed and available to use, some of the most popular languages today are Python, C, C++, Java, JavaScript PHP, R, HTML, Ruby, and Scala, among others. When you master one language, switching to another is relatively easy because the underlying logic is the same. Therefore, it is only a matter of learning a new syntax - the rules that structure a language.
Script
A Python script is a file containing a set of instructions that perform a specific task.
You can recognize them by specific file extensions - just like you would recognize a Microsoft Word file by its extension .doc
or .docx
, you can spot a Python script by extension .py
.
To actually perform the task we programmed, we have to execute a script using Python.
Debugging
Finding errors in computer code is often refered to as catching bugs, and fixing them as fixing bugs or debugging. Debugging, therefore, means correcting errors in the code.
Historical note
Term debugging is attributed to Admiral Grace Hopper, who worked at Harvard University in the 1940s. Her co-workers found a moth stuck in the computer, thereby impeding its functioning. She famously remarked that the system needs debugging.
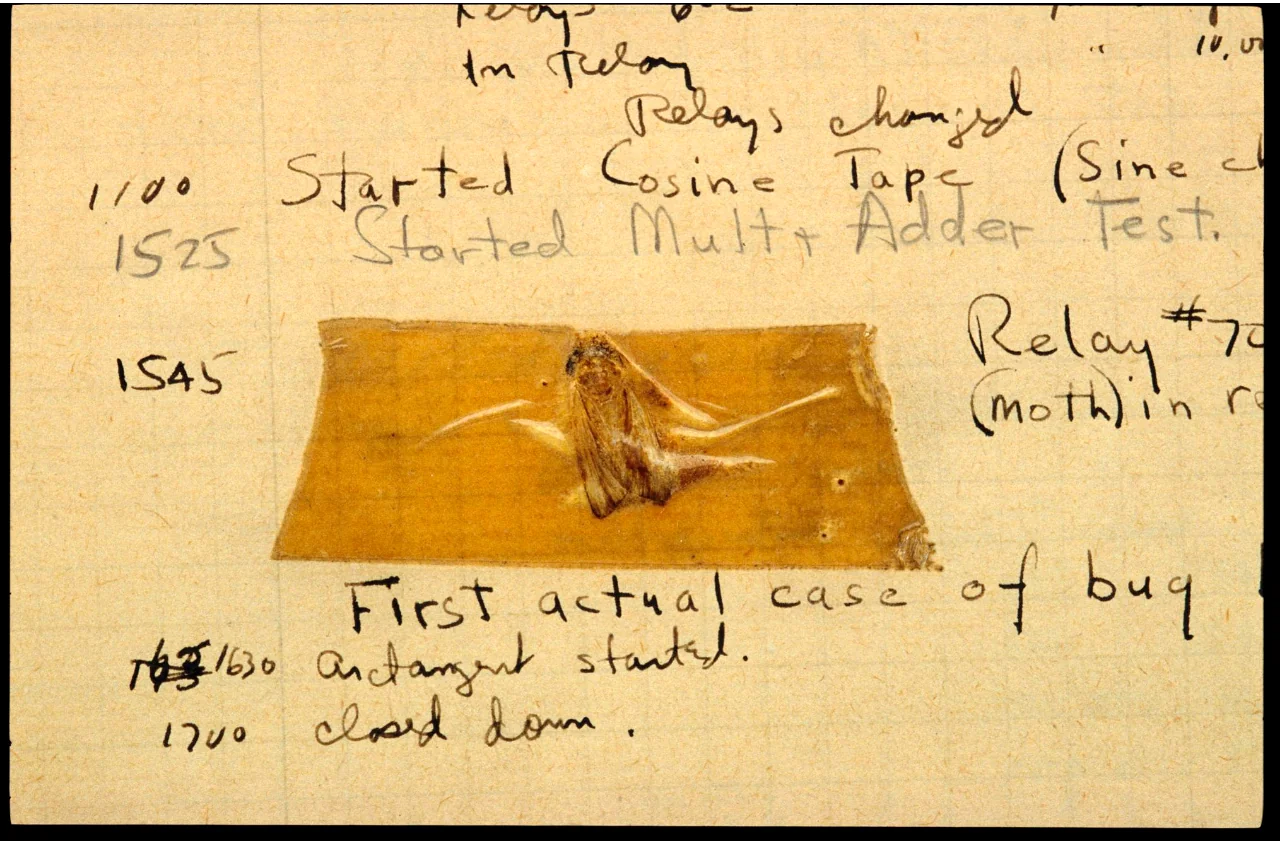
Fig. 1.4 The first case of a computer bug (photo source, image from the public domain).#
Package / Library
Packages are building blocks of programming. They consist of reusable pieces of code, so that you do not have to program everything from scratch (and thereby “reinvent the wheel”). Sometimes we refer to published packages as libraries. Examples of Python libraries are NumPy for scientific computing and Matplotlib for visualization.
Function
A Python function is a piece of reusable code that performs a specific task. You can either define functions by yourself (in your code) or use predefined ones, e.g., functions that come as part of Python packages.
Package management
Like many things in computer world, Python packages get improved over time. As a consequence, new versions of packages are regularly released. Because a part of one package can depend on a part of another package, it is very important to keep track of used packages, their specific versions, and their dependencies. There are specialized tools for Python package management - pip and conda - which help us to avoid package version conflicts and ensure that the packages we use are compatible.
Environment
A Python environment is a setup in which a Python script is executed. For instance, if you use functions from the NumPy package in your code, (a specific version of) NumPy has to be present in the environment. Because packages can depend on each other, and because details of functions can change between versions, your code may stop working (“break”) upon package update. It is, therefore, good practice to work within environments that contain specific versions of packages needed in your code. There are also different types of Python environments: system environment, conda environment, virtual environment, and containerized environment.
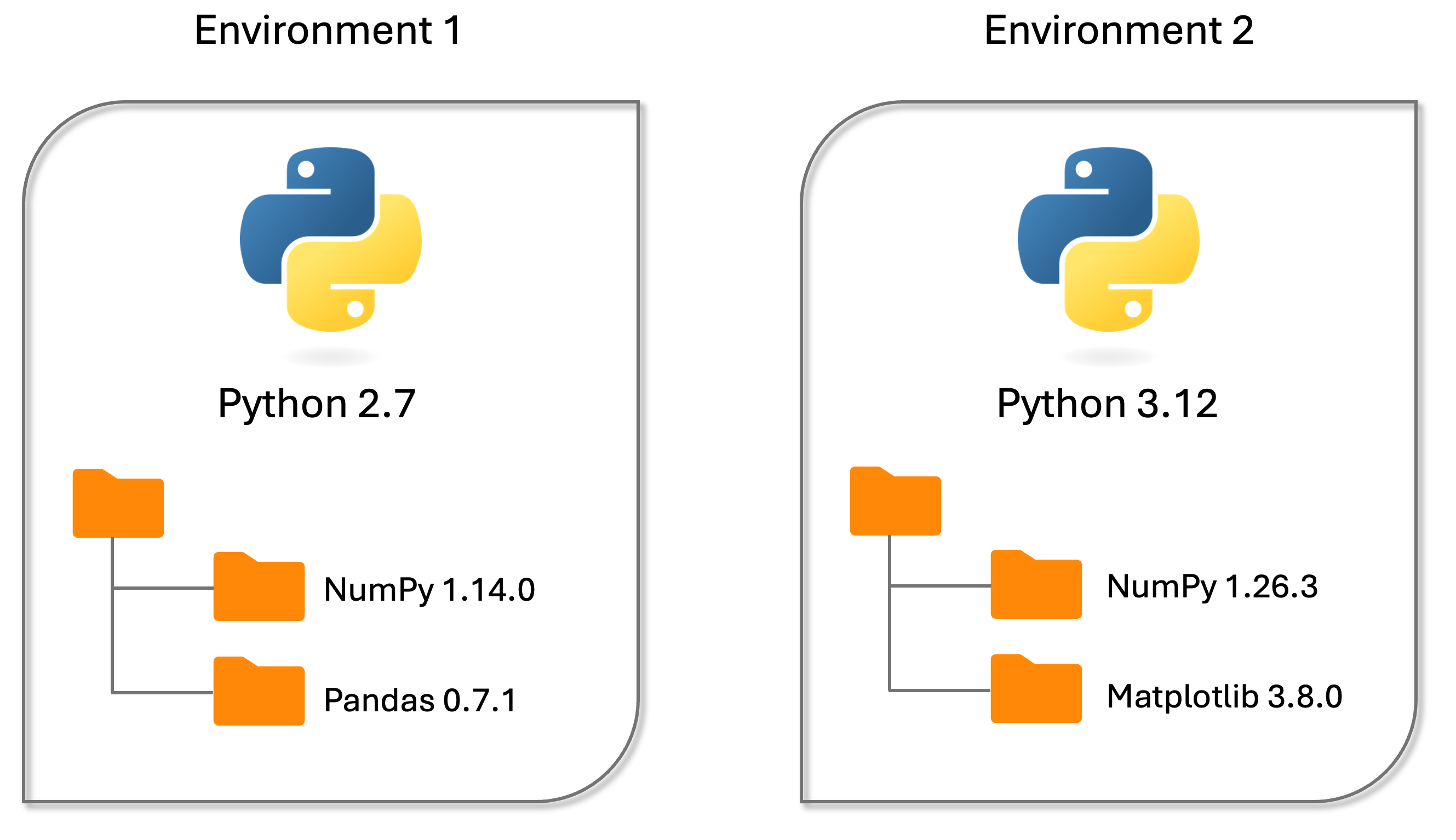
Fig. 1.5 Illustration of two separate Python environments, each containing a different version of Python and different packages.#
Version control
In the context of programming, version control is the management of changes in computer code. It is extremely useful, as it allows for tracking changes (seeing everything that was modified between two commits), effective collaboration on code, etc. The most popular version control program is called Git.
Terminal, command line interface, and BASH shell
The Terminal is an application that allows communication with the operating system using command line interface (CLI), which is useful for saving time and automating repetitive tasks. Unlike applications with graphical user interfaces (GUIs) - which contain buttons, sliders, and other interactive elements - using the terminal requires us to enter lines of code (commands) in CLI in order to perform tasks. These commands are then executed by a shell, which is a user interface to the operating system. Different shells can be used to run commands. A default shell program used by macOS and Linux is BASH (Bourne-Again SHell), while Windows uses PowerShell.
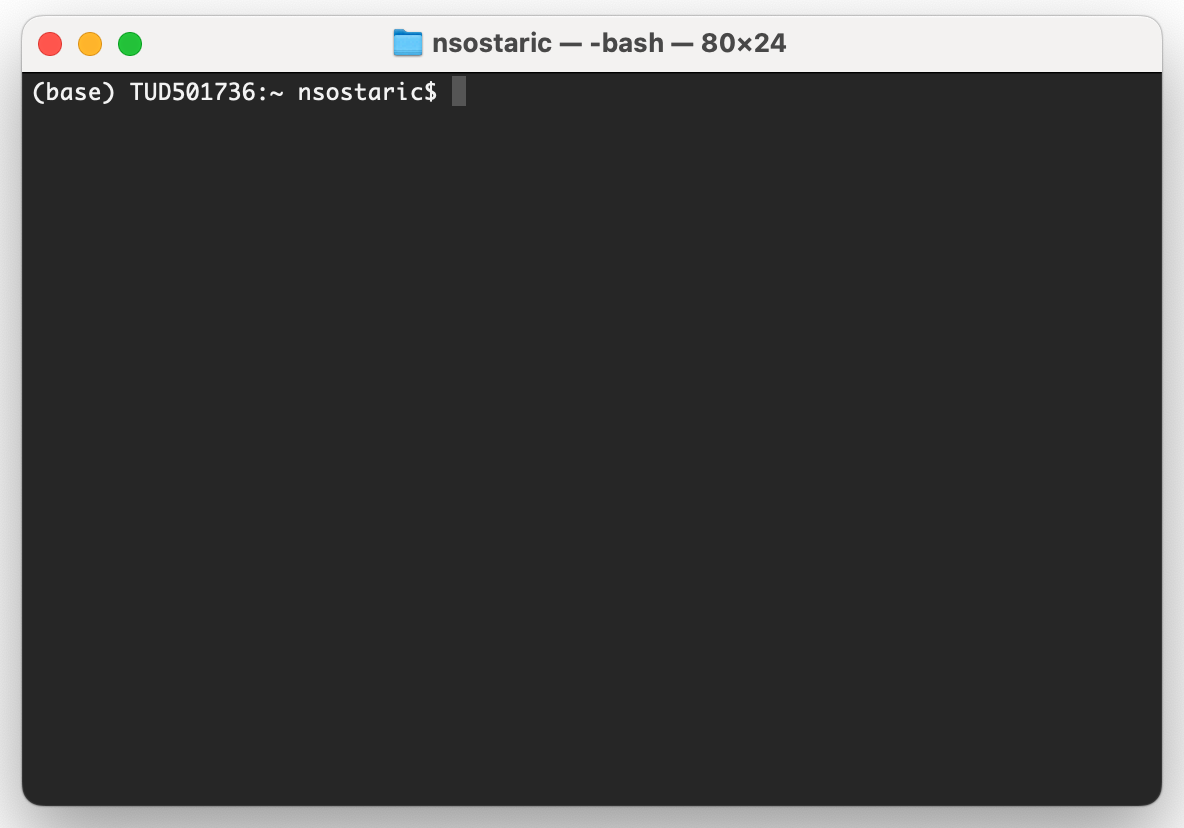
Fig. 1.6 Terminal on macOS.#